Stabilized Orthogonal Factorizations
1.Conditioning of linear algebra problems
Recall that the relative condition number of a mathematical problem
characterizes the amplification by of
perturbations in its argument
Linear combination. The basic operation of linear
combination ,
,
seen as the problem
has the condition number
The matrix norm property can be used to obtain
leading to
where is the condition number of the matrix
. If
is of full rank with ,
the 2-norm condition number is given by the ratio of largest to smallest
singular values.
By convention, if is singular, the
condition number .
Coordinate transformation. For
of full rank, the coordinates of vector
expressed in the basis can be
transformed its coordinates
in the basis by solving the linear
system ,
with the solution
(so written formally, even though the inverse is almost never explicitly
computed). This is simply another linear combination of the columns of
,
hence the problem ,
again has a condition number bounded by the condition number of the
matrix .
Operator perturbation. Instead of changing the input data as
above, the linear mapping represented by the matrix
might itself be perturbed. Two mathematical problems may now be
formulated:
-
For fixed .
Perturbation of the input induces
perturbation of in order for to be kept fixed
Using ,
and assuming that
is negligible gives
hence the relative condition number is
For all above linear algebra problems the condition number is bounded by
the associated matrix condition number. Unitary matrices
have , and define an
orthonormal basis for .
A rank-deficient matrix
has , and corresponds
to a linearly dependent vector set . The behavior of many numerical approximation
procedures based upon linear combinations is determined by condition
number of the basis set.
Monomial basis with uniform
sampling. Sampling the monomial basis on interval
at ,
leads to the Vandermonde matrix
an extremely ill-conditioned matrix (Fig. ). This can readily be
surmised from the example ,
, in
which case for large the last columns
of become ever more colinear to the
same vector.
Series expansions based on the monomials such as the Taylor series
are highly sensitive to pertubations, small changes in lead to
large changes in the coordinates .
∴ |
function Vandermonde(a,b,m)
t=LinRange(a,b,m); v=ones(m,1); V=copy(v)
for j=2:m
v = v .* t; V=[V v]
end
return V
end; |
Monomial basis with Chebyshev
sampling. Changing the sampling so that points are clustered
towards the interval endpoints reduces the condition number at fixed
number of sampling points , but the same
limiting behavior for large is
obtained.
∴ |
function VandermondeC(m)
δ=π/(2*m); ϴ=LinRange(δ,π-δ,m)
t=cos.(ϴ)
v=ones(m,1); V=copy(v)
for j=2:m
v = v .* t; V=[V v]
end
return V
end; |
Triangular basis with uniform
sampling. -factorization
of the monomial basis leads to a different family of polynomials,
known as a triangular basis
where are known as the nodes of the system. These
can be chosen to uniformly sample an interval. As to be expected,
applying a sequence of non-unitary linear transformations onto an
ill-conditioned basis yields even worse conditioning.
∴ |
function Triangular(a,b,m)
x=LinRange(a,b,m); T=ones(m,1); Tj=copy(T); t=copy(x)
for j=2:m
Tj = Tj .* (t .- x[j-1]); T=[T Tj]
end
return T
end; |
Triangular basis with Chebyshev
sampling. Adopting Chebyshev sampling ameliorates the
conditioning, but only marginally.
∴ |
function TriangularC(m)
δ=π/(2*m); ϴ=LinRange(δ,π-δ,m)
x=cos.(ϴ); T=ones(m,1); Tj=copy(T); t=copy(x)
for j=2:m
Tj = Tj .* (t .- x[j-1]); T=[T Tj]
end
return T
end; |
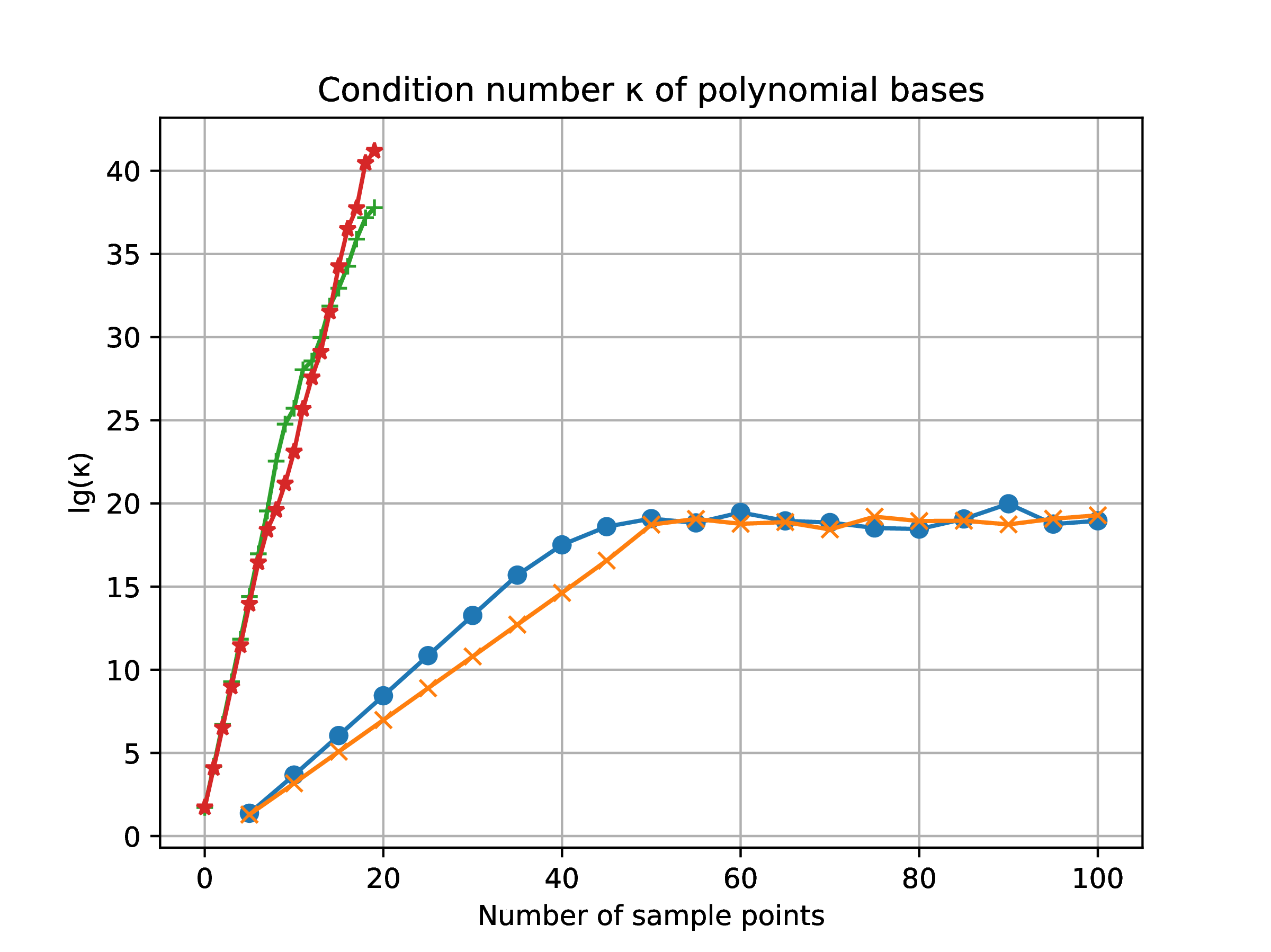 |
|
Figure 1. Monomial basis with: (o) uniform
sampling, (x) Chebyshev sampling. Triangular basis with: (+)
uniform sampling, (*) Chebyshev sampling.
|
∴ |
mr=5:5:100; κVDMU=log10.(cond.(Vandermonde.(-1,1,mr))); |
∴ |
κVDMC=log10.(cond.(VandermondeC.(mr))); |
∴ |
κTU=log10.(cond.(Triangular.(-1,1,mr))); |
∴ |
κTC=log10.(cond.(TriangularC.(mr))); |
∴ |
plot(x,κVDMU,"o-",x,κVDMC,"x-",κTU,"+-",κTC,"*-"); |
∴ |
grid("on"); title("Condition number κ of polynomial bases"); |
∴ |
xlabel("Number of sample points"); ylabel("lg(κ)"); |
∴ |
pre="/home/student/courses/MATH661/images/"; |
∴ |
savefig(pre*"PolyBasesCondNr.eps"); |
2.Orthogonal factorization through Householder
reflectors
The Gram-Schmidt procedure constructs an orthogonal factorization by
linear combinations of the column vectors of ,
,
In exact arithmetic by construction, and ,
but the sequence of multiplications with
might amplify perturbations in (due
for example to floating point representation errors or inherent
uncertainty in knowledge of ). The
problem
has condition number
and numerical experimentation (Fig. 2) readily exhibits
large condition numbers.
An alternative approach is to obtain an orthogonal factorization through
unitary transformations
Unitary transformations do not modify vector 2-norms or relative
orientations
and are hence said to be isometric. In Euclidean space reflections and
rotations are isometric.
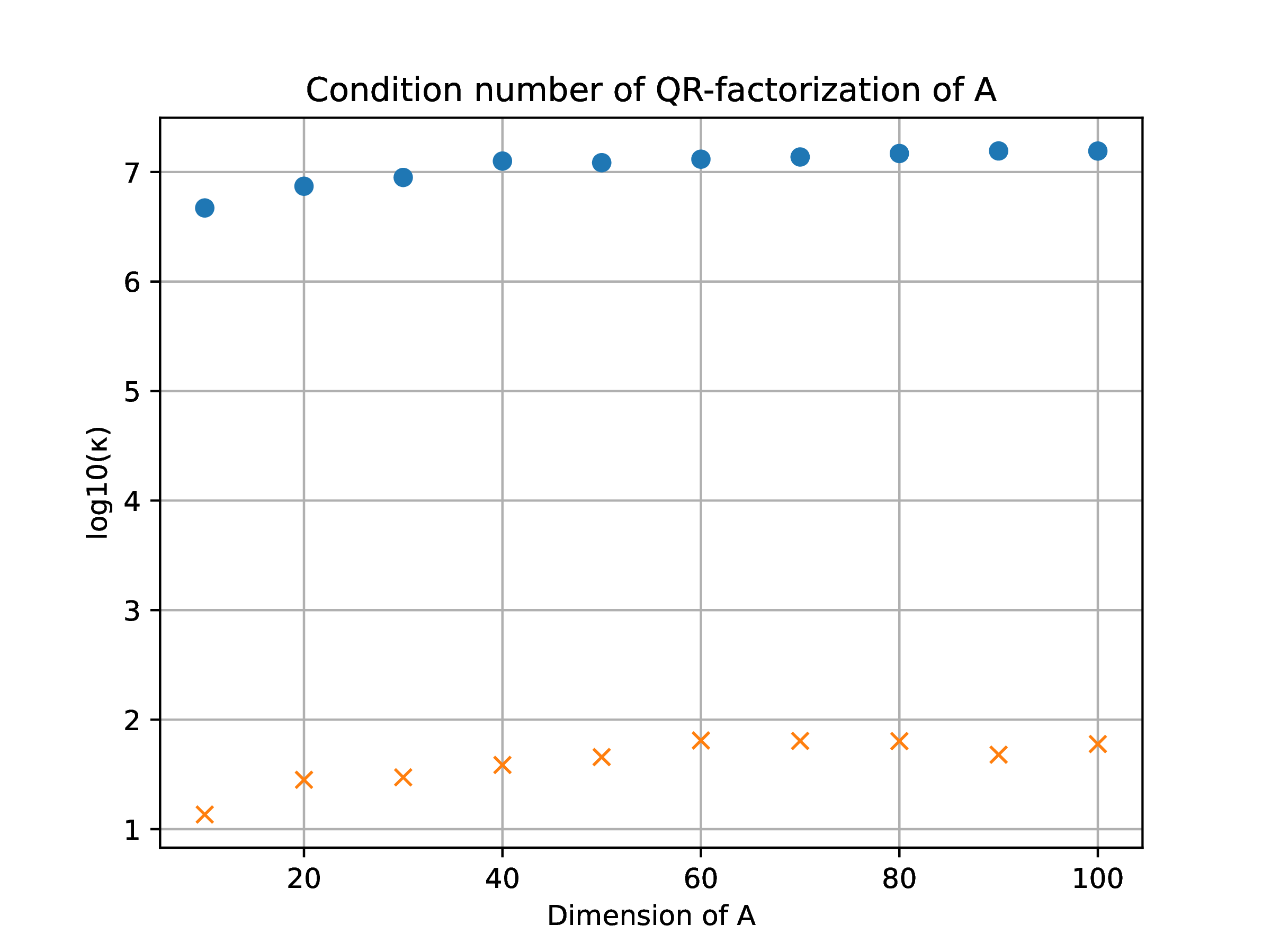 |
|
Figure 2. -conditioning:
(o) modified Gram-Schmidt, (x) Householder.
|
Estimation of the -condition
number by numerical experimentation: generate
random perturbations of a matrix, compute the factorization of each
perturbed matrix, and choose the maximum encountered value as .
∴ |
function mgs(A)
m,n=size(A); Q=copy(A); R=zeros(n,n)
for i=1:n
R[i,i]=sqrt(Q[:,i]'*Q[:,i])
if (R[i,i]<eps())
break
end
Q[:,i]=Q[:,i]/R[i,i]
for j=i+1:n
R[i,j]=Q[:,i]'*A[:,j]
Q[:,j]=Q[:,j]-R[i,j]*Q[:,i]
end
end
return Q,R
end; |
∴ |
function QRcondGS(N,A,ε)
Q,R=mgs(A); κ=1; normA=norm(A); normR=norm(R)
for k=1:N
δA=ε*randn(size(A))
δF=qr(A+δA); δQ=Array(δF.Q)-Q; δR=Array(δF.R)-R
κ = max(κ, (norm(δQ)+norm(δR)/normR)*normA/norm(δA) )
end
return κ
end; |
∴ |
mr=10:10:100; κQRGS = log10.(QRcondGS.(100,randn.(mr,mr),1.0e-6)); |
∴ |
x=collect(mr); clf(); plot(x,κQRGS,"o"); |
∴ |
function QRcond(N,A,ε)
F=qr(A); Q=Array(F.Q); R=Array(F.R)
κ=1; normA=norm(A); normR=norm(R)
for k=1:N
δA=ε*randn(size(A))
δF=qr(A+δA); δQ=Array(δF.Q)-Q; δR=Array(δF.R)-R
κ = max(κ, (norm(δQ)+norm(δR)/normR)*normA/norm(δA) )
end
return κ
end; |
∴ |
κQR = log10.(QRcond.(100,randn.(mr,mr),1.0e-6)); |
∴ |
x=collect(mr); plot(x,κQR,"x"); |
∴ |
grid("on"); title("Condition number of QR-factorization of A"); |
∴ |
xlabel("Dimension of A"); ylabel("log10(κ)"); |
∴ |
savefig(pre*"QRcond.eps"); |
Construction of an isometric reflection transformation suitable for a
factorization is represented in Fig. 3. Let vector
represent the portion of the
column from the diagonal downwards in stage
of reduction of
to upper triangular form
The next stage of in reduction to upper triangular form is the isometric
transformation of into ,
with
the unit vector along the first direction. With , , the
projection of onto the span of , is
and its complementary projector onto
is
The reflector transforming into
is obtained by doubling the above displacements, and is known as a
Householder reflector
Of the two possibilities ,
the choice
avoids loss of floating accuracy .
For ,
.
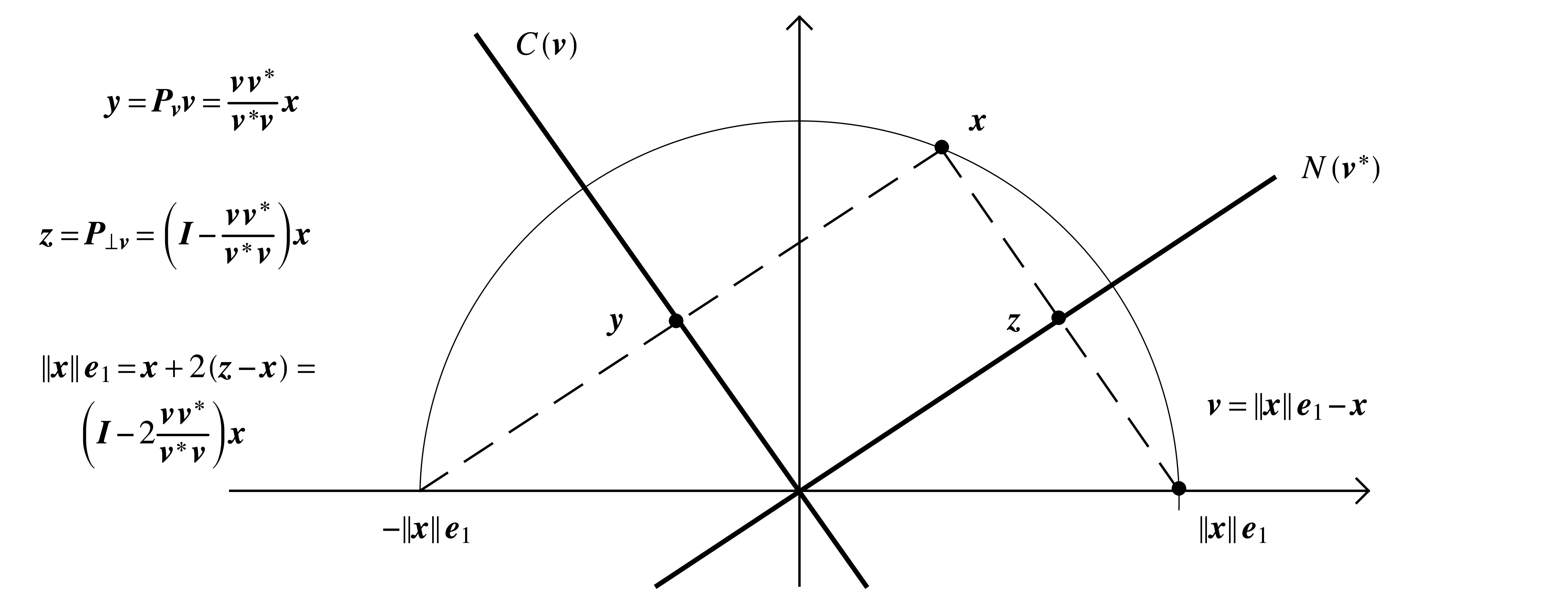 |
|
Figure 3. Geometry of Householder
reflector
|
The resulting Householder -factorization
is given
|
∴ |
function HouseholderQR(A)
m,n=size(A)
Q=zeros(m,n); R=copy(A)
for k=1:n
x=R[k:m,k]
e1=zeros(size(x)); e1[1]=1
v=sign(x[1])*norm(x)*e1+x
q=v/norm(v); Q[k:m,k]=q
for j=k:n
aj=R[k:m,j]; c=2*q'*aj
R[k:m,j]=aj.-c*q
end
end
return Q,R
end; |
|
Note that the above implementation does not return the
matrix, but rather the
reflectors from which can be
reconstructed if needed. Usually though, the
matrix itself is not required, but rather the product
which can readily be evaluated as .
The Householder reflector algorithm is typically the default procedure
in -factorizations
implemented in software systems, and as seen in (Fig. 2),
leads to much better conditioning.
3.Orthogonal factorization through Given
rotators
An alternative approach to orthogonal factorization utilizes isometric
rotation transformations of the form
with the rotation angle chosen to
nullify the subdiagonal element ,
Composition of repeated rotations can be organized to lead to an upper
triangular matrix
Whereas Householder reflectors work on entire vectors, Givens rotators
nullify individual subdiagonal elements. For full matrices Householder
reflectors typically require fewer floating point operations, but the
special structure of a sparse matrix is better exploited by use of
Givens rotators.
;
|
∴ |
function GivensQR(A)
m,n=size(A)
Q=zeros(m,n); R=copy(A)
for k=1:n
for i=k+1:m
θ = atan(-R[i,k],R[k,k]); Q[i,k]=
c = cos(θ); s = sin(θ)
for j=k:n
u = R[k,j]; v = R[i,j]
R[k,j]=c*u-s*v
R[i,j]=s*u+c*v
end
end
end
return Q,R
end; |
|
As in the Householder implementation the above implementation returns
data to reconstruct if needed.